Null Checking in C#
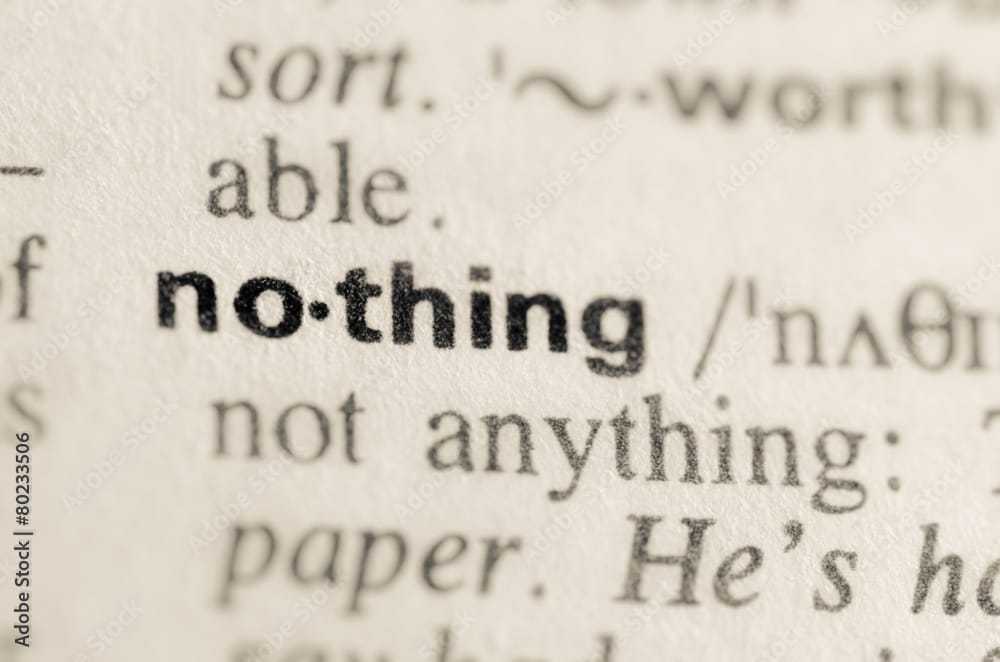
Just a quick post today on the subject of null.
Checking for null in the bane of many programming languages. And while there are some clever techniques and design patterns that can help minimise the need to do this, it’s inevitable that you’ll end up needing to check for null.
So lets look at the low-level basics of checking for null, and what features later versions of the C# language offers.
The == operator
The age-old method of checking for null is pretty straight forward, and hardly worth creating a blog post about.
MyObject foo = null;
if (foo == null)
{
// throw some helpful exception
}
Of course, you can invert the logic as well:
MyObject foo = null;
if (foo != null)
{
// do something useful
}
I still catch myself using this method without thinking, as it natural to want to compare the value of one variable to another. There is a subtle problem with this approach however:
The Equals operator of any object can be overridden internally. And in doing so, the behaviour of foo == null
can be changed. Imagine what a bug in that Equals method might do to null checks in your application?!
So, there is a safer method.
The ‘is’ keyword
Since C# version 7, it is possible to use a new keyword to check for null and/or the type of the object.
MyObject foo = null;
if (foo is null)
{
// throw a helpful exception
}
This not only avoids the equality operator override issue above, but also just reads better.
There is another catch however. It’s not that simple to invert this logic. If you only wanted to execute a block of code if something was NOT null, it would look like this:
MyObject foo = null;
if (!(foo is null))
{
// do something useful instead
}
Suddenly we’ve lost that nice code readability. And I think this is possibly the reason use of this new keyword was initially limited. However, Microsoft eventually came to the rescue with an update to this.
The ‘is not’ keyword
C# version 9 finally introduced the ‘is not’ keyword, which allows us to return to the nice, readable format:
MyObject foo = null;
if (foo is not null)
{
// do something useful
}
More adventures with the ‘is’ keyword
The ‘is’ keyword is actually far more versatile than a simple null check. It also allows for some basic combining of a null check, cast and assignment in a single line of code.
Take the following example, where we have two constructs. One an interface, the other a concrete class that implements the interface.
The if() statement is now able to:
1. Check if the value of foo
is null.
2. Check if foo
is of the type MySuperClass
3. And if it is, cast foo
to that type, and assign it to a variable called superFoo
public interface IMyInterface
{
...
}
public class MySuperClass : IMyInterface
{
...
}
IMyInterface foo = new MySuperClass();
if (foo is MySuperClass superFoo)
{
// do something useful
}
In fact, the is
keyword allows us to do all sorts of fancy pattern matching as well. Take a look at this method from the Microsoft docs that demonstrates the `is` keyword being used with the property pattern:
static bool IsFirstFridayOfOctober(DateTime date) =>
date is { Month: 10, Day: <=7, DayOfWeek: DayOfWeek.Friday };
So as you can see, there are multiple benefits for learning and switching to using the is
keyword for null and type checking.
Further Reading
The is
operator is well documented in the C# Language Reference docs at Microsoft:
is operator – C# reference | Microsoft Docs